Mastering JavaScript
About the book
JavaScript is one of the most powerful programming languages on the web! With it, you can build highly dynamic websites and apps (including both frontend and backend development!).
Have you ever wanted to learn Angular, React, Vue or Node.js? A solid understanding of JavaScript fundamentals will make picking up JS frameworks a breeze!
We cover it all from basic Syntax & Structure, Variables and Data Types to the Document Object Model (the DOM), Arrays, Functions, Loops, and Objects. We also dive into advanced topics such as working with APIs, Async and Defer, Promises, Regex operations & more!
Mastering JavaScript will benefit your web development skills enormously - and it's in very high demand professionally, today the average salary for JavaScript developers are some of the highest in tech!
Available in PDF, EPUB & MOBI. đ
* Secured payment with Gumroad.
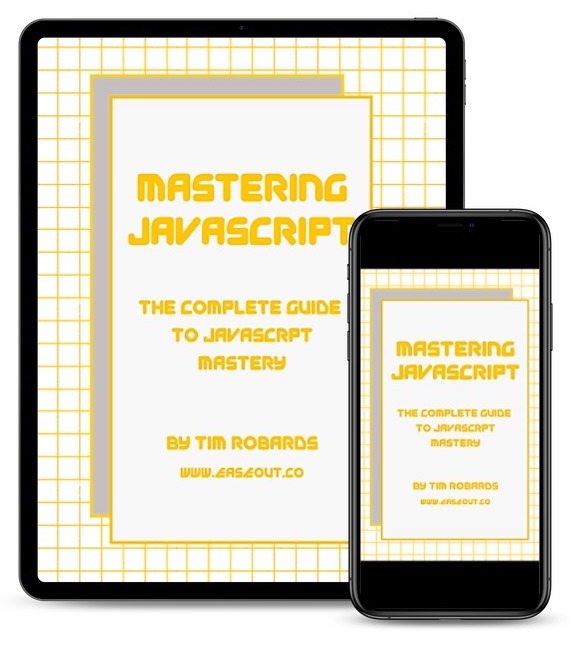
Table of Contents
- Introducing JavaScript
- How to add JavaScript to a webpage
- How to add inline JavaScript
- How to link to external JavaScript
- Syntax and Structure
- Values
- Camel Case
- Unicode
- Semicolons
- Indentation
- White space
- Commenting
- Identifiers
- Case sensitivity
- Reserved words
- JavaScript Operators
- JavaScript Expressions
- Variables, Scope and Hoisting
- What are Variables?
- Naming Variables
- Difference between var, let and const
- Variable scope
- Hoisting
- Constants
- Data Types
- What are Data Types?
- Converting Data Types
- Converting Values to Strings
- Converting Values to Numbers
- Converting Values to Booleans
- The Basics of JavaScript Strings
- Creating Strings
- Concatenation
- Syntax rules
- Working with Strings
- The Anatomy of a String
- Converting Strings into Numbers
- An Introduction to the DOM
- What is the DOM?
- The DOM Tree and Nodes
- Accessing DOM Elements
- getElementById()
- getElementsByClassName()
- getElementsByTagName()
- Query Selectors
- Traversing the DOM
- Root Nodes
- Parent Nodes
- Child Nodes
- Sibling Nodes
- Making changes to the DOM
- Creating New Nodes
- Inserting Nodes into the DOM
- Removing Nodes from the DOM
- How to modify DOM Nodes
- Modifying Attributes
- Modifying Classes
- Modifying Styles
- Understanding Events
- What are Event Handlers and Listeners?
- Inline Event Handlers
- Event Handler Properties
- Event Listeners
- Common Events
- Event Objects
- Mastering Arrays
- Creating an Array
- Looping over an Array
- Adding to an Array
- Removing from an Array
- Array indexes/li>
- Additional Array Operations
- Mastering Objects
- Creating an Object
- Understanding the syntax
- Updating Objects
- Understanding 'this' in an Object
- Using Conditionals
- if/else statements
- else if
- Comparison Operators
- Nesting
- Logical Operators
- Switch statements
- The Ternary Operator
- Mastering Loops
- The for loop
- The do ... while statement
- Labelled, break and continue
- for in and for of
- Mastering Functions
- Defining Functions
- Function Expressions
- Calling Functions
- Function Scope
- Nesting and Closures
- Arguements Object
- Function Parameters
- Arrow Functions
- Predefined Functions
- What are Classes?
- Class Declairations
- Class Expressions
- Constructors
- Other useful Class properties
- Understanding APIs
- APIs in JavaScript
- How do API's work?
- Fetching data from a server
- The old way
- The solution: AJAX
- Creating an AJAX request
- XMLHttpRequest
- Fetch
- An Introduction to REST APIs
- What is a REST API?
- RESTful Architecture
- REST in action
- Working with REST data
- Promises
- Why use Promises?
- Async and Defer
- What are the methods?
- Why use each method?
- The 'this' keyword
- What is 'this'?
- Examples of execution contexts
- Understanding Regex
- A very basic regex pattern
- Why use Regular Expressions?
- Creating a Regular Expression
- Regular expression methods
- The power of Regex
- Flags
- Character Groups
- Conclusion
Technical Details
- 226 pages
- Available in PDF, EPUB & MOBI Formats
- Updated for 2024!